
Introduction – C++ Beginners Guide – Loops and Iteration
C++ Beginners Guide – Loops and Iteration is a guide to using loops in C++. This article’s principles apply to all programming languages; however, the syntax will change between different languages.
For Loop
For loops start at one index value then increment or decrement that value with each loop. In each loop the value is checked against a condition. If the condition has not been met, the loop continues. In this example the loop starts at 0 and keeps going until the value exceeds 10 and then exits the loop.
For Loop Diagram
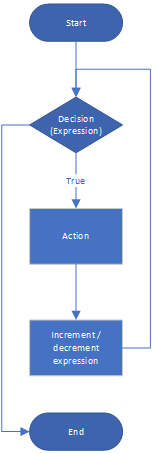
For Loop Example Code
#include <iostream> using namespace std; int main() { int maxValue = 10; int value = 0; int totalOfValues = 0; for (value; value <= maxValue; value++) { totalOfValues += value; } cout << "total of all values from 0 to 10 inclusive is: " << totalOfValues << endl; return 0; }
While Loop
While loops check to see if a condition is true or false. If its true the action is carried out and then the condition is checked again. Only when the exit condition is matched will the loop break.
While Loop Diagram
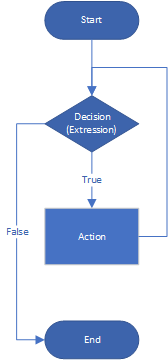
While Loop Example Code
#include <iostream> using namespace std; int main() { int maxValue = 10; int value = 0; int totalOfValues = 0; while (value <= maxValue) { totalOfValues+=value; value++; } cout << "total of all values from 0 to 10 inclusive is: " << totalOfValues << endl; return 0; }
Do While Loop – – C++ Beginners Guide – Loops and Iteration
Do while loops work in almost the same way as the while loop above, however, the action will be performed one time regardless of the condition set. The condition is checked after the action has been performed.
Do While Loop Diagram
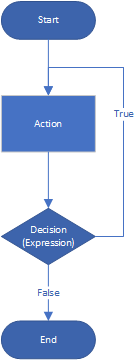
Do While Loop Example Code
#include <iostream> using namespace std; int main() { int maxValue = 10; int value = 0; int totalOfValues = 0; do { totalOfValues+=value; value++; } while (value <= 10); cout << "total of all values from 0 to 10 inclusive is: " << totalOfValues << endl; return 0; }
Range Based For Loop – Same principle as a for each loop in other languages.
Range based for loops will iterate over every member of an array or similar data structure. It will exit once the last element in the array has been processed. This is exactly the same principle as the for each loop in C#.
Range Based For Loop Diagram
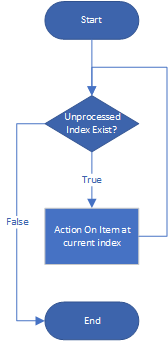
Range Based For Loop Example Code
#include <iostream> using namespace std; int main() { int numbers [11] = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; int value = 0; int totalOfValues = 0; for (int value : numbers) { totalOfValues+=value; } cout << "total of all values from 0 to 10 inclusive is: " << totalOfValues << endl; return 0; }
Exit A loop – – C++ Beginners Guide – Loops and Iteration
Loops can exit based on conditionals such as the examples above. Additionally, values can be tested to make sure they conform to certain types. The following loop has two possible exit clauses:
#include <iostream> using namespace std; int main() { double inputVariable = -1; while(inputVariable) { cout << "enter a negative number;"; cin >> inputVariable; if(inputVariable > 0.0) { break; } } cout << "A positive number or a non numeric value has been entered, exiting"<< endl; return 0; }
Firstly if a non-numeric value such as the character ‘h’ is entered, the loop will exit. Secondly, if the value entered is not negative, the loop will also exit using the “break” keyword. It is worth noting that if the loop is nested (a loop inside a loop), only the loop where the beak sits will exit.
Jumping to the next loop iteration – – C++ Beginners Guide – Loops and Iteration
In this example rather than exiting the loop if a positive value is entered, it will skip to the next loop iteration. This will ignore all positive entries and keep looping until a non-numeric value is entered. To jump to the next loop iteration the “continue” keyword is used.
#include <iostream> using namespace std; int main() { double inputVariable = -1; while(inputVariable) { cout << "enter a negative number;"; cin >> inputVariable; if(inputVariable > 0.0) { cout << "A positive value was entered, ignoring" << endl; continue; } } cout << "A non numeric value has been entered, exiting"<< endl; return 0; }
2 thoughts on “C++ Beginners Guide – Loops and Iteration”