C# Beginners – Lists And Arrays; Covers the basics of using lists and arrays in C#. This tutorial is part of a series. Before attempting this tutorial, you should have chosen an IDE and be comfortable with it. Additionally, you should be familiar with the essential data types and conditional statements for making a selection. If this is not the case, please see previous tutorials in this series. There is a video version of this tutorial at the end of the post or by clicking here.
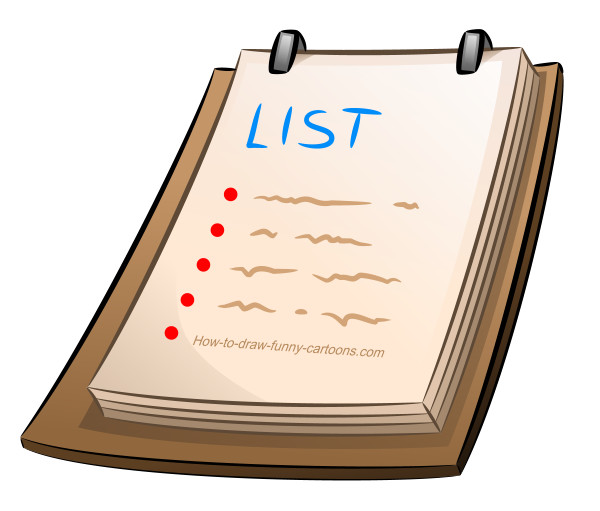
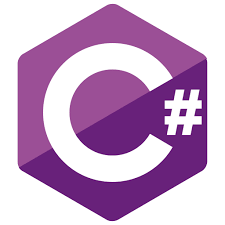
What are Lists and Arrays?
Lists and arrays are very similar and work similarly to lists found in other walks of life, such as a shopping lists. An array is essentially a list with a predefined number of spaces. Comparatively, a List grows and contracts dynamically. The syntax for defining lists and arrays is slightly different.
The critical difference between Lists in C# and lists outside of programming is that lists and arrays can only hold a single data type.
Basic Syntax – C# Beginners – Lists And Arrays
The equal sign acts as an assignment operator. Everything before the equal sign creates the variable, and everything after the equal sign sets the content of the variable. Additionally, each element in a list or array has an index number. The first element in a list or array in C# is at index zero
Element | word 1 | word 2 | word 3 | word 4 | word 5 |
Index | 0 | 1 | 2 | 3 | 4 |
Arrays
Initially, it sets the type, followed by a pair of square brackets to signify that it is an array. Firstly, it sets the variable’s name, and an equal sign follows. Secondly, it says that this is a new array of length five. Finally, inside the curly brackets, the content is specified.
int [] numberArray = new int[5]{5, 1, 10, 16, 20}; string[] stringArray = new string[5]{ "word1", "word2", "word3", "word4", "word5" };
Declaring the array without adding content so it can be added later is possible.
int [] numberArray = new int[5]; string[] stringArray = new string[5];
Lists
Lists are defined slightly differently; initially, the fact it is a list is stated, followed by the data type for the list and then the variable’s name. Next, it says that this is a new list and defines the content of the list.
List<int> numberList = new List<int>() { 99, 54, 33, 200, 1 }; List<string> stringList = new List<string>() { "word1", "word2", "word3", "word4", "word5" };
Like with arrays, we can define a list without giving it any content.
List<int> numberList = new List<int>() {}; List<string> stringList = new List<string>() {};
Get and Set Element Contents in Arrays and Lists – C# Beginners – Lists And Arrays
Every element in an Array or List has an index number. We use the index number to get the element’s contents or to update the element’s contents. The syntax and behaviour for this is identical with both Lists and Arrays.
The example below defines the same list and array as before. Then, there are a couple of console write lines to display the content of that element. Note that square brackets are used to set the index number.
List<string> stringList = new List<string>() { "word1", "word2", "word3", "word4", "word5" }; string[] stringArray = new string[5]{ "word1", "word2", "word3", "word4", "word5" }; Console.WriteLine(stringArray[3]); Console.WriteLine(stringList[3]);
Running the code above will give the following output:
word4
word4
Setting the content is similar. The example below still uses the square brackets and index numbers. However, the equal sign is the assignment operator to set the value.
It starts with the short program above for reading the contents in the first four lines. Then, it sets new values and rereads the same element.
List<string> stringList = new List<string>() { "word1", "word2", "word3", "word4", "word5" }; string[] stringArray = new string[5]{ "word1", "word2", "word3", "word4", "word5" }; Console.WriteLine(stringArray[3]); Console.WriteLine(stringList[3]); stringArray[3] = "a new string"; stringList[3] = "a new string too"; Console.WriteLine(stringArray[3]); Console.WriteLine(stringList[3]);
The output from this program will be:
word4
word4
a new string
a new string too
Notice that even though the console writeline accesses the same element before and after assigning the value, the output is different because the element’s content is different.
Add and Remove List Elements – C# Beginners – Lists And Arrays
This section applies only to lists. Arrays have a fixed size that can not change. With lists, it is possible to create new elements or remove them. The size of the list will then change dynamically. New list elements can be added to the end or inserted at a specified index position.
Creating new list elements
This example starts with an empty list and then adds an element before outputting the element’s value to the console.
List<string> stringList = new List<string>() {}; stringList.Add("Word1"); Console.WriteLine(stringList[0]);
The program above will output the following:
Word1
The following example starts the same way, then inserts a new value at index zero, which increases the index number for all elements that were at the specified index or that follow it. Inside the brackets of the insert section are two parts separated by a comma. The first is the index number, and the second is the value to be inserted into the element.
List<string> stringList = new List<string>() {}; stringList.Add("Word1"); Console.WriteLine("Index 0: " + stringList[0]); stringList.Insert(0, "New string"); Console.WriteLine("Index 0: " + stringList[0]); Console.WriteLine("Index 1: " + stringList[1]);
The output from the above code will be:
Index 0: Word1
Index 0: New string
Index 1: Word1
Remove list elements
There are two ways to remove a list element: remove the element at a specified index or remove the first element that matches a specified value.
The example below creates a list with 5 elements and then outputs the content. Next, it removes the contents of a specified index and outputs them again. Finally, it removes the first element that matches the specified content and outputs once more.
List<string> stringList = new List<string>() { "word1", "word2", "word3", "word4", "word5" }; Console.WriteLine("Initial List"); Console.WriteLine("Index 0: " + stringList[0]); Console.WriteLine("Index 1: " + stringList[1]); Console.WriteLine("Index 2: " + stringList[2]); Console.WriteLine("Index 3: " + stringList[3]); Console.WriteLine("Index 4: " + stringList[4]); Console.WriteLine("\nRemoving index 1, list now:"); stringList.RemoveAt(1); Console.WriteLine("Index 0: " + stringList[0]); Console.WriteLine("Index 1: " + stringList[1]); Console.WriteLine("Index 2: " + stringList[2]); Console.WriteLine("Index 3: " + stringList[3]); Console.WriteLine("\nRemoving the first \"Word3\", list now:"); stringList.Remove("Word3"); Console.WriteLine("Index 0: " + stringList[0]); Console.WriteLine("Index 1: " + stringList[1]); Console.WriteLine("Index 2: " + stringList[2]);
The program above will give the following output:
Removing index 1, list now:
Index 0: word1
Index 1: word3
Index 2: word4
Index 3: word5
Removing the first "Word3", list now:
Index 0: word1
Index 1: word3
Index 2: word4
Note how each time the number of indexes output is reduced. This is because the list after each removal becomes smaller.
Array and List size (how many elements they have)
Getting the size of lists and arrays is often an essential element of programming that will become apparent when we look at loops. Arrays are a fixed size, and lists are dynamic, but both have mechanisms for getting their size. The syntax is slightly different, but the idea is the same.
Array Size
The example below creates an array. In this example, the size of the array is not specified; instead, it is set by the number of elements in it initially. Then, the number of elements is counted and stored in an integer variable. Finally, the value of the count is output to the console.
string [] arrayStrings = new string[] {"Word1", "Word2", "Word3", "Word4", "Word5"}; int arraySize = arrayStrings.Length; Console.WriteLine(arraySize.ToString());
The code above will output:
5
List Size
The example below creates a list with five elements and then defines an integer with the number of elements in the list. Finally, it outputs the value in the integer variable.
List<string> listStrings = new List<string>() { "Word1", "Word2", "Word3", "Word4", "Word5" }; int listSize = listStrings.Count; Console.WriteLine(listSize.ToString());
The code above will output
5
Video Version – C# Beginners – Lists And Arrays
Related Articles – C# Beginners – Lists And Arrays
- C# Beginners – IDE Choice
- C# Beginners – Using Rider
- C# Beginners – Visual Studio
- C# Beginners – Using Vs-Code
- C# Beginners – Start Coding
- C# Beginners Variables & Types
- C# Beginners – User Input
- C# Beginners – If Else Selection
- C# Beginners – Switch Statement
- C# Beginners – Try/Catch/Finally
- C# Beginners – Loops
6 thoughts on “C# Beginners – Lists And Arrays”