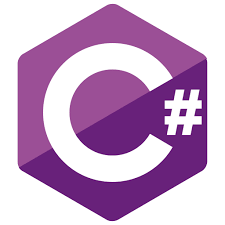
Introduction – C# Beginners Variables & Types
C# Beginners Variables & Types; this post looks at what a variable is and some of the data types available. This is the first post in the series that will focus primarily on the code rather than the IDE. It assumes you have chosen an IDE, can create a new C# solution and run a program. If not, please review one of the earlier tutorials in this C# Beginners Series.
What Is A Variable
A variable is, at a technical level, an area in memory. However, it is easier to think of a variable as a box with a label on it. Like a box on a shelf, the designation identifies it. But, unlike a box in the physical world, a variable can only hold one type of item, or more accurately, one kind of data.
What is a Data Type
Data Types are the way programmers identify different kinds of data. For example, in the physical world, we might locate wood as a typical building material and bricks as another. Programming works with the same principle. Therefore, we need to identify the type of data the variable stores.
C# has many data types; a complete list is available from the Microsoft Reference Guide; however, this post will focus on strings, integers and doubles.
Strings, Integers and Doubles
- Strings: text
- Integers (int): whole numbers
- Doubles: decimal numbers
Data Type Limits
The description of integers and doubles above is very simplistic. However, it is a deliberate choice for a beginner’s guide. Anyone interested in the limits of these data types in C# should see the Characteristics Of the integral types and Floating-point numeric types in the Microsoft documentation.
Declaring Variables In C# – C# Beginners Variables & Types
When we declare a variable, we reserve an area in memory to store some data. The program uses the variable name to identify the memory location. To declare a variable in C#, we must first state the data type and then give the variable a name. In the example below, ‘string’ is the data type and ‘myStringVariable’ is the name.
string myStringVariable;
We can make other types of variables in similar ways.
string myStringVariable; double myDoubleVariable; int myIntegerVariable;
Assigning Values To Variables
Declaring variable reserves a space in memory to store data. Assigning a value puts the data into the reserved memory. Like putting an apple into a box with a label on it that reads ‘apples’. We assign data to a variable in programming using the ‘=’ sign. E.g.
string myStringVariable = "Hi, i am Ms Payne";
Notice that there are quotation marks around the text. With numbers, we do not do this.
int myDoubleVariable = 5; double myIntegerVariable = 5.5;
About the underlines in the IDE
If you put this code into an IDE, it will underline the variable names. E.g.

The warning is because noting uses the variable. When you first declare and assign a variable, this is normal. However, knowing it can be deleted if your program was finished with an unused variable is helpful. Hovering over the underline will show further information.
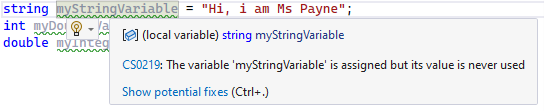
Using Variables
After data has been assigned to a variable, you can reaccess it using the variable name. In the code below, first, I declare a variable of type string called ‘myName’ and then assign ‘Ms Payne’ to the variable. Next, I use Console.WriteLine to output the contents of ‘myName’ to the Console.
string myName = "Ms Payne"; Console.WriteLine(myName);
Notice how when you use the variable, the underline warning about it never being used vanishes.
Experiment with the two code examples below. Run the program to see the difference in the out.
string myName = "Ms Payne"; Console.WriteLine(myName);
Output
Ms Payne
string myName = "Ms Payne"; Console.WriteLine("myName");
Output
myName
The first of the code snippets above outputs the contents of the variable ‘myName’, and the second outputs a literal string ‘myName’. The difference between these two bits of code is the presence of quotation marks in the Console.WriteLine(“myName”).
Can’t Mix Data Types – C# Beginners Variables & Types
At the start, I stated that a variable can only contain a single data type. This means we can not mix them either. Take a look at the image below.
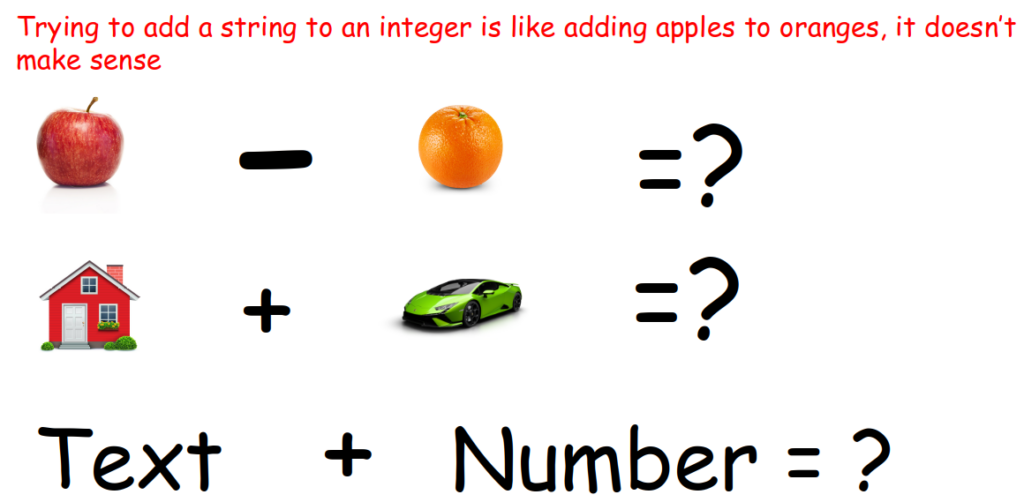
Automatic Casting (Conversion)
Sometimes there are automatic conversions between data types if the IDE and compiler know a sensible outcome. Try the following two code snippets and compare the differences in the IDE. Try running them too.
string myStringVariable = "Hi, i am Ms Payne"; double myDoubleVariable = 5.5; string myStringVariable2 = myStringVariable + myDoubleVariable; Console.WriteLine(myStringVariable2);
string myStringVariable = "Hi, i am Ms Payne"; double myDoubleVariable = 5.5; double myDoubleVariable2 = myStringVariable + myDoubleVariable; Console.WriteLine(myDoubleVariable2);
Hopefully, you will spot the instant difference in the IDE
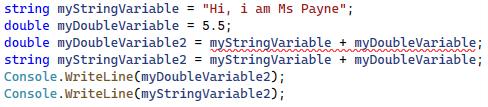
This is because C# can represent a number as text. But, it can not represent text as a number sensibly.
First, in the top example, C# casts (converts) the number to text, then concatenates (joins) the two bits of text together. Second, the bottom example above cannot convert the text to a number, so it underlines this to tell you it is not possible. If you run this program, it will crash and give an error message.
String Concatenation (Joining Strings)
Strings can be combined (concatenated) using the ‘+’ operator. E.g.
string firstName = "Sara"; string lastName = "Payne"; string fullName = firstName + lastName; Console.WriteLine(fullName);
Output
SaraPayne
Run the code above. Did you get the output you expected? Concatenating strings does not add spaces; space must be added manually. Try again with the following code.
string firstName = "Sara"; string lastName = "Payne"; string fullName = firstName + " " + lastName; Console.WriteLine(fullName);
Output
Sara Payne
The plus operator is the only one that can be used with strings. As stated above, this is because it is concatenating them rather than doing any kind of arithmetic on them. Attempting to use any other operator will result in errors. E.g.
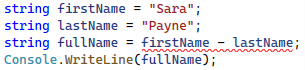
Integer and Double Arithmetic
Programming Arithmetic Operators
It is worth discussing the symbols used for those new to programming. The characters we see in maths are:
- ÷ (divide)
- × (multiply)
- + (add)
- – (subtract)
In programming, the symbols for add and subtract are the same; however, divide and multiply are different.
- / (divide)
- * (multiply)
- + (add)
- – (subtract)
Integer and double arithmetic throw up a few surprises for new programmers. Similarly to strings, several conversions will happen automatically. However, others will require you as the programmer to explicitly state the conversion.
Adding two integers together or two doubles gives the results you might expect. E.g.
int number1 = 5; int number2 = 5; int number3 = number1 + number2; Console.WriteLine(number3); double dec1 = 5.5; double dec2 = 5.1; double dec3 = dec1 + dec2; Console.WriteLine(dec3);
Output
10
10.6
However, if you try to combine integers and doubles, things become a little more involved. Try the code below in your IDE.
int number1 = 5; double number2 = 5.5; int number4 = number1 + number2; double number5 = number1 + number2;
You should see the third line is underlined.
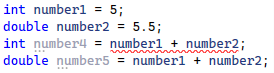
This is because C# can convert an integer (whole number) to decimal without any loss of accuracy. However, it can not convert a decimal value to an integer without losing accuracy. To get around this, as a programmer, if we really want an integer result, we must cast the result to an integer. E.g.
int number1 = 5; double number2 = 5.5; int result = (int)(number1 + number2); Console.WriteLine(result);
Output
10
It does not mathematically round when we cast a decimal number to an integer. Instead, it ignores the decimal part as if it doesn’t exist. So, for example, in the following code, after the cast, the integer value is always 10.
double dec1 = 10.1; int num1 = (int)dec1; Console.WriteLine(num1); double dec2 = 10.5; int num2 = (int)dec2; Console.WriteLine(num2); double dec3 = 10.9; int num3 = (int)dec3; Console.WriteLine(num3);
Output
10
10
10
Integer Division
Dividing an integer by an integer is interesting for a related but different reason. Sometimes if an integer is divided by an integer, it will give a whole number answer. For example, 10 divided by 2 is 5. However, 10 divided by 4 does not result in a whole number.
Try the following code in your IDE to observe the behaviour.
int num1 = 10; int num2 = 4; int intResult = num1/num2; double dblResult = num1 / num2; double dblResult2 = (double)num1 / (double)num2; Console.WriteLine("Integer Division Result: " + intResult); Console.WriteLine("Cast Double After Result: " + dblResult); Console.WriteLine("Cast Double Before Result: " + dblResult2);
Notice that no IDE warnings are given when dividing an integer by an integer and asking for an integer result.
The differences between Visual Studio and Rider regarding this code are worth noting.
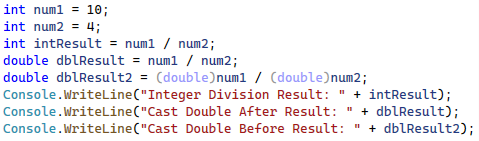
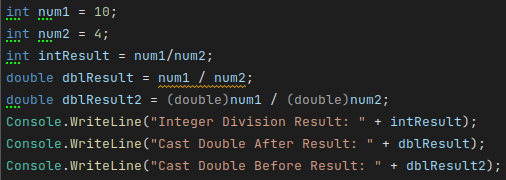
Output
Integer Division Result: 2
Cast Double After Result: 2
Cast Double Before Result: 2.5
Rider warns the programmer about a possible loss of accuracy on line 4, but the visual studio does not. Differently, neither warns about a loss of precision for line 3. It is essential to be aware of this when working with a division.
Firstly, line 3 divides the integers, and the result is given as an integer.
Secondly, line 4 divides the integers, and the result is given as an integer and then cast to a double.
Thirdly, line 5 casts each integer to a double before dividing the two doubles and giving a double result.
Video Version – C# Beginners Variables & Types
Related Articles – C# Beginners Variables & Types
- C# Beginners Series Introduction
- C# Beginners – IDE Choice
- C# Beginners – Visual Studio
- C# Beginners – Using Vs-Code
- C# Beginners – Using Rider
- C# Beginners – Start Coding
- C# Beginners – User Input
- C# Beginners – If Else Selection
- C# Beginners – Switch Statement
- C# Beginners – Try/Catch/Finally
- C# Beginners – Lists And Arrays
- C# Beginners – Loops
8 thoughts on “C# Beginners Variables & Types”