Introduction
Python Beginners – Lists; lists are precisely what you might imagine from the name. They are a list of data; however, the data in a list must all be the same data type. A video version of this tutorial is available at the bottom of the blog or by clicking here.
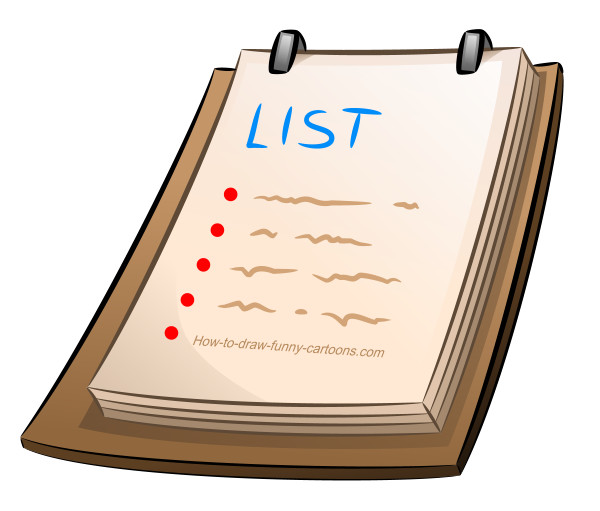
The first example below is a list of strings. Lists are defined inside square brackets [], and each element in the list is separated by a comma. See the example below.
colours = ["red", "green", "blue"]
The code above declares a variable called ‘colours’ and then assigns a list of type ‘string’ that contains three elements. The elements are:
- red
- green
- blue
List Indexes – Python Beginners – Lists
Each element in a list has an index number. The first index number in a Python list is zero. It is possible to access any index number individually. E.g.
Element | red | green | blue | yellow | brown | lilac | orange | white | black | pink |
Index Number | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
The code below is an example of this using indexes. It defines a list of strings and then prints each element of the list by using the element index number.
colours = ["red", "green", "blue"] print(colours[0]) print(colours[1]) print(colours[1])
Running this program will output three lines.
red
green
blue
Experiment with the code; try one print statement at a time and change the index number.
List Data Types
Lists can contain any data type. For example, the code below adds two more lists, one of type ‘integer’ and the other of type ‘float’.
strings = ["red", "green", "blue"] integers = [2, 5, 6, -10] floats = [2.5, -8.4, -9.1, 3.78]
The variable names do not need to include the type information. I have just done it here to clarify the list type. All lists contain elements inside square brackets []. Each element in the list is separated by a comma. With lists of strings, each string has quotes around it. Lists can also contain variables, but each element must have the same type. E.g.
number1 = 5 number2 = 20 number3 = -300 number4 = 1000 numbers = [number3, number1, number4, number2] name1 = "sally" name2 = "tom" name3 = "Emma" name4 = "Lei" names = [name4, name3, name2, name1]
Two things are worth noting in the above code. First, when adding variables of type string into a list, there is no need to add quotation marks. Adding them would add the string inside the quotes instead of the variable. Second is that the order of the elements is not essential. Items can be added to a list in any order.
Finally, it is possible to mix variables with explicitly stated data, again, so long as the data type matches. See the examples below.
number1 = 5 number2 = 20 number3 = -300 number4 = 1000 numbers = [5, number3, number1, ,-99, number4, number2, 2412] name1 = "sally" name2 = "tom" name3 = "Emma" name4 = "Lei" names = [name4, "Ben", name3, name2, "Clair", name1]
Adding And Removing List Elements
So far, the lists in this post have all been defined in the code; however, elements can be added and removed.
Appending Items To Lists
In the example below, a list with a single element is defined. Then a second element is appended to the end. There is also a print statement before and after the append line. This helps to see what happens when the program executes.
names = ["Dan"] print(names) names.append("Sally") print(names)
Insert List Elements – Python Beginners – Lists
Append adds a new list element to the end of the list. Differently, insert lets us specify the index to insert the element. The example below starts with the program above and then inserts a new element at index 1. Any existing elements at that index are moved up one index position.
names = ["Dan"] print(names) names.append("Sally") print(names) names.insert(1, "Chris") print(names)
Remove Specified Item – By Content
Items can be removed if they match the specified content. In the example below, a list is defined with three string elements. Then an element is removed based on the content added to the remove like. Like the previous examples, there are print statements to show what happens before and after the removal process.
names = ["Sara", "Dean", "Kate"] print(names) names.remove("Sara") print(names)
It is important to note that this will only remove the first element it finds that matches the remove statement. Try the code below. It is the same as above, except an extra element, “Sara”, is in the initial list.
names = ["Sara", "Dean", "Sara", "Kate"] print(names) names.remove("Sara") print(names)
Remove (Pop) List Items By Index
It is possible to remove list elements with their index number. All elements in the list with a higher index number have their index numbers reduced by 1. The example below defines a list and then pops the element at index 1. There are print statements before and after the removal.
names = ["Sara", "Dean", "Kate"] print(names) names.pop(1) print(names)
Remove All List Elements (clear the list)
You can remove all list elements with the clear command. In the example below, the code defines a list and then clears it. . Similarly to the previous examples, there are print statements before and after the clear.
names = ["Sara", "Dean", "Kate"] print(names) names.clear() print(names)
9 thoughts on “Python Beginners – Lists”