Python Beginners – Selection; selection is another name for making a decision. All programming language decisions are based on true or false statements. This post focuses on the selection’s coding side; another post will detail binary logic.
Let us start with a simple example program. This is the example in the IDE choice post.
Selection Code – If/Else
name = input("your name please") if name == "Ms Payne": print("you are " + name) else: print("You are not Ms Payne")
Code Explanation – Python Beginners – Selection
The program above does the following:
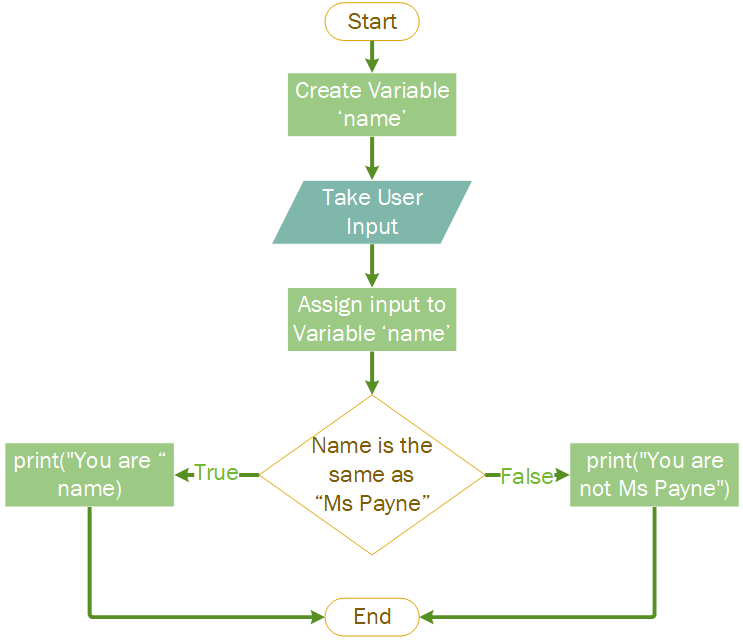
- Define (create) a variable called ‘name’.
- Take a user input.
- Assign the user input to the variable ‘name’.
- Is the variable ‘name’ content the same as “Ms Payne”?
- True:
- print(“You are”, name)
- False:
- print(“You are not Ms Payne”
- True:
Video Demonstration of the selection at work.
Code Blocks and Syntax
- Take a user input.
- Assign the user input to the variable ‘name’.
- Is the variable ‘name’ content precisely the same as “Ms Payne”?
- True:
- print(“You are”, name)
- False:
- print(“You are not Ms Payne”
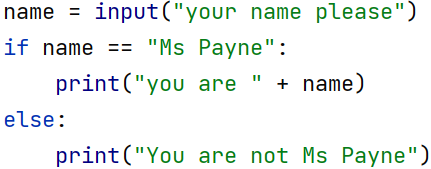
Both the If and Else code blocks contain a single print statement. Code blocks are represented by an indentation in Python. A code block can have multiple lines. E.g.
name = input("your name please") if name == "Ms Payne": print("you are " + name) print("Well done") else: print("You are not Ms Payne") print("Better luck next time")
The above code works exactly the same way, but each code block now has two lines of code.
Types Of Comparison – Python Beginners – Selection
Strings
Strings can be compared to see if they are equivalent to another string. This will return true if they are, meaning the condition is met, and the If statement code will run. If the statement is false (the strings do not precisely match), then the else block will execute.
Numerical Data Types – Integers and Floats
Numerical Data Can be compared in more ways
Comparison Type | Operator Symbol |
Equivalent to | == |
Less than | < |
Greater than | > |
Less than or equal to | =< |
Greater than or equal to | >= |
Numerical Data Type Comparison Examples
Comparison Example | Result (True or False) |
Ms Payne == Ms Payne | True |
ms Payne == Ms Payne | False |
3 == 3 (3 is equivalent to 3) | True |
3 > 3 (3 is greater than 3) | False |
3 < 3 (3 is less than 3) | False |
3 =< 3 (3 is less than or equal to 3) | True |
3 > = 3 (3 is greater than or equal to 3) | True |
2 < = 3 (2 is less than or equal to 3) | True |
4 <= 3 (4 is less than or equal to 3) | False |
2 >= 3 (2 is greater than or equal to 3) | False |
4 >= 3 (4 is greater than or equal to 3) | True |
Boolean Logic Basics – Python Beginners – Selection
String comparison Example
Every decision comes down to a statement being True or False, as in the table above. True and False values in programming and called boolean or bool. The code example above can be written another way.,
name = input("your name please") nameCheck = bool(name == "Ms Payne") if nameCheck == True: print("you are " + name) else: print("You are not Ms Payne")
The code above can be simplified by removing the ‘== True’ bit and leaving the boolean variable ‘namCheck’.
name = input("your name please") nameCheck = bool(name == "Ms Payne") if nameCheck == True: print("you are " + name) else: print("You are not Ms Payne")
Video Example of Basic Boolean Logic – String Comparison
Numerical Comparison Example
number1 = 5 number2 = 10 lessThan = bool(number1 < number2) if lessThan: print(number1, " is less than ", number2) else: print(number1, " is not less than ", number2)
The code above will output ‘5 is less than 10’. But it is usually written differently. See the example below.
number1 = 5 number2 = 10 if number1 < number2: print(number1, " is less than ", number2) else: print(number1, " is not less than ", number2)
Both programs do precisely the same thing; they evaluate whether the statement ‘5 is less than 10’ is True or False. If it is True, the code in the If block is executed; otherwise, the Else block is executed. All the comparison operators from the table above are available.
If / Elif / Else – Python Beginners – Selection
The If / Else block can be extended to include an elif statement (else if).
If statement 1 is True, then do the following:
Else if statement 2 is True, then do the following:
Else do:
number1 = 5 number2 = 10 if number1 < number2: print(number1, " is less than ", number2) elif number1 == number2: print(number1, " is equivalent to ", number2) else: print(number1, " is not less than ", number2)
9 thoughts on “Python Beginners – Selection”