Python Beginners – Loops; Looping is a fundamental part of almost every program. This tutorial assumes that you already understand the basics of lists and selection. If this is not the case, please do the Python Beginners – Selection and Python Beginners – Lists tutorials before attempting this one. This tutorial covers all forms of iterative loops in Python (for, range and while), but it does not cover recursive functions. I will cover recursive functions after covering functions. There is a video version at the end of this post, also available here.
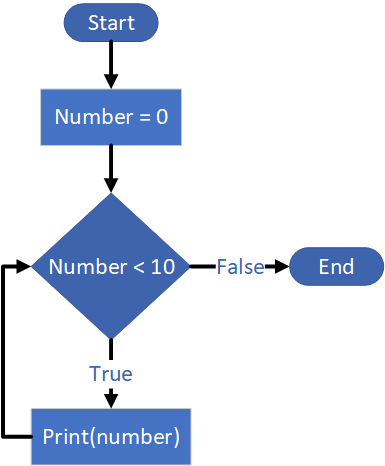
Looping typically works one of three ways: an operation is done a specific number of times, carried out on all the elements in a list, or, lastly, until a particular condition is met. Before going into loops, learning another feature of lists is useful. This is the ability to get the length of a list programmatically.
List Length (len)
The ‘len’ function in Python allows us to find out how many elements there are in a list. Remember, however, that counting starts at 0. So in the example below, the length is 4, the first index is 0, and the last index is 3.
listNames = ["Craig", "Emma", "Sam", "Alex"] listLength = len(listNames) print(listLength)
Index | 0 | 1 | 2 | 3 |
Element | Craig | Emma | Sam | Alex |
While Loop – Python Beginners – Loops
While loops will run until a specific condition is met. For example, a while loop might run until a value hits a particular value. However, it is essential to remember that, like with selection, what is actually happening is a statement that is either True or False.
E,g,
listNames = ["Craig", "Emma", "Sam", "Alex"] print("Full List:", listNames) listLength = len(listNames) print("List Length:", listLength) index = 0 while index < 4: print("Index: ", index, "is", listNames[index]) index += 1
Let us start by breaking this program down, line by line
- Prints the complete list of names in the way seen previously
- Declares a variable of name ‘listNames’ and then assigns four strings as elements in a list to the variable
- Declares a new variable called ‘listLength’ and then assigns the length of the list to it, using the ‘len’ function to calculate it.
- Prints the length of the list.
- Declares a new variable called ‘index and assigns the value of 0.
- Declares a while loop and sets the condition, so it keeps running WHILE the value of the variable index is less than 4
- Prints the current index number and the contents of the variable list names in the element number specified by the value in the variable index.
- Increases the value of the index by 1
- The loop then runs again if the condition is met.
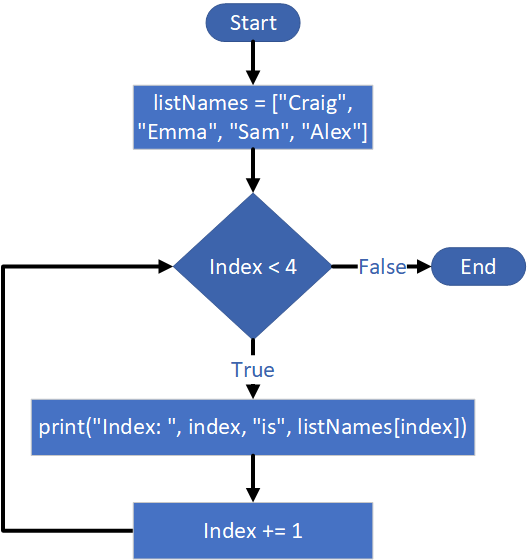
There are many possible ways to write a while loop that does the same thing as the code above. However, one crucial way is by using ‘len’ from above. The code below is the same, except for using ‘len’ to calculate the list length. This change means we don’t need to know how big it will be when we write the loop.
Implied boolean value in a while loop.
In the example below, ‘while index < listLength’ is actually making a statement that evaluates to True or False. The correct way of reading that is ‘index is less than the value stored in listLength’, True or False.
listNames = ["Craig", "Emma", "Sam", "Alex"] print("Full List:", listNames) listLength = len(listNames) print("List Length:", listLength) index = 0 while index < listLength: print("Index: ", index, "is", listNames[index]) index += 1
Explicitly Declared Boolean
Similarly to selection (if/else), this code can explicitly set the boolean instead. E.g.
listNames = ["Craig", "Emma", "Sam", "Alex"] print("Full List:", listNames) listLength = len(listNames) print("List Length:", listLength) index = 0 indexInRange = bool(index < listLength ) while indexInRange: print("Index: ", index, "is", listNames[index]) index += 1 indexInRange = bool(index < listLength )
Exiting a loop with a break statement
Writing a loop that will run forever (infinite loop) is also possible. It is imperative to set a break condition in such a case. Break conditions exit the loop from an if statement. Making the loop ‘while True’ means it will keep looping forever. E.g.
listNames = ["Craig", "Emma", "Sam", "Alex"] print("Full List:", listNames) listLength = len(listNames) print("List Length:", listLength) index = 0 while True: print("Index: ", index, "is", listNames[index]) index += 1 if index >= listLength: break
In the code above, it is worth taking a close look at the ‘if’ statement. The operator is the reverse of the previous two examples. It runs the ‘break’ statement if the value of ‘index’ is greater than or equal to the value of ‘listLength’. I could have said, “if the value of ‘index’ is not less than listLength”, but I have not yet covered that type of boolean logic. Consequently, I felt that the operator reversal was easier to understand.
For Loop – Python Beginners – Loops
Python’s ‘for’ loop works differently from some other languages. C# users will be familiar with a ‘foreach’ loop. Foreach is an excellent way to think of a ‘for’ loop in Python. For each element ‘in’ a ‘list’, do something. E.g.
listNames = ["Craig", "Emma", "Sam", "Alex"] print("Full List:", listNames) listLength = len(listNames) for name in listNames: print("Name:", name)
The first three lines in the code above are familiar by this point. However, the ‘for’ loop warrants a little explanation. Think of it as follows:
- Declare a for loop
- Declare a variable called ‘name’.
- Assign the value of the first element in ‘listNames’ to the variable ‘name’.
- Print the contents of the variable ‘name’.
- Assign the value in the next element to the variable name and keep looping until no elements are left in the list.
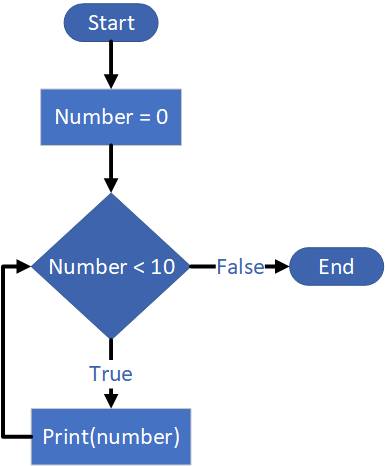
Range Loop – Python Beginners – Loops
Range loops are still ‘for’ loops; however, instead of integrating through each element in a list, they iterate from one value to another in a range. Python will default assume that counting starts at zero unless specified and finish before the range end. E.g.
listNames = ["Craig", "Emma", "Sam", "Alex"] for index in range (4): print("Index:", index, "Name:", listNames[index])
The code above outputs the elements at indexes 0, 1, 2 and 3. Like with the while loop, the range can be a variable. E.g.
listNames = ["Craig", "Emma", "Sam", "Alex"] listLength = len(listNames) for index in range (listLength): print("Index:", index, "Name:", listNames[index])
It is also possible to set the start index. The example below sets the start index to 1, meaning index 0 is not included in the output.
listNames = ["Craig", "Emma", "Sam", "Alex"] listLength = len(listNames) for index in range (1, listLength): print("Index:", index, "Name:", listNames[index])
Increasing in increments other than 1 is done by adding a third value to the range loop. In the example below, only the first and third elements are printed. The loop starts on index 0, then increases by 2 and prints index 2, then exits because adding another two goes beyond the last index in the list.
listNames = ["Craig", "Emma", "Sam", "Alex"] listLength = len(listNames) for index in range (1, listLength): print("Index:", index, "Name:", listNames[index])
Finally, a range loop does not need to work with a list; it can be a range of numbers for mathematical purposes. E.g.
for number in range (0, 10, 2): print(number, "*", number, "=", number*number)
In the code above, each even number starting at 0 and less than 10 is squared and printed.
Strings And Loops
It is possible to loop through a ‘string’ character by character. However, it is essential to note that Python does have a data type, ‘char’. Characters are treated as strings. E.g.
While Loop and Strings
name = "Ms Payne" stringLength = len(name) index = 0 while index < stringLength: print (name[index]) index +=1
For Loop and Strings
name = "Ms Payne" for character in name: print(character)
Range Loop and Strings
name = "Ms Payne" stringLength = len(name) index = 0 for index in range (stringLength): print(name[index])
9 thoughts on “Python Beginners – Loops”